utilizing BenchmarkDotNet.Attributes; utilizing BenchmarkDotNet.Operating;
To complete the process, execute the following command in your console:
dotnet run -p SplitStringsPerformanceBenchmarkDemo.csproj -c Release
The outcomes of the executed benchmarks are illustrated in Exhibit 2 below:
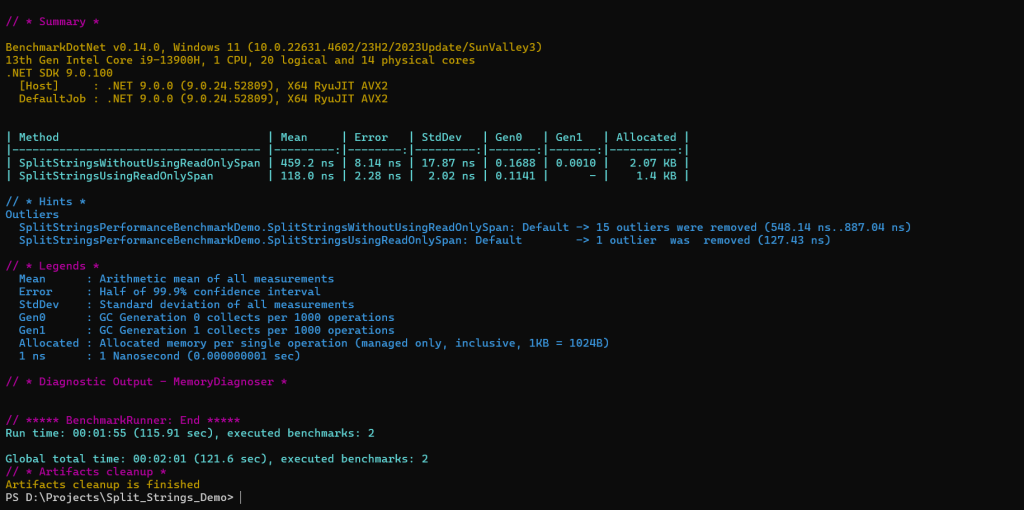
Determine 2. Evaluating the ReadOnlySpan<char>.Break up() and String.Break up() strategies utilizing BenchmarkDotNet.
IDG
As you’ll be able to see from the benchmarking leads to Determine 2, the ReadOnlySpan<char>.Break up() methodology performs considerably higher in comparison with the String.Break up() methodology. The efficiency information displayed pertains to a single execution of each strategy. When repeatedly running benchmark strategies across multiple iterations, subtle efficiency fluctuations become more pronounced.
The ReadOnlySpan<char>.Break up() methodology is a sooner, allocation-free various to the String.Break up() methodology in C#. Span-based strategies in C# prove to be significantly more environmentally friendly, minimizing the need for Gen 0 and Gen 1 garbage collection compared to traditional String class approaches. By scaling back their reminiscence footprint and reducing waste collection overheads substantially, they achieve significant cost savings.