In the trendy world of Python development, securely managing configuration settings and sensitive information is crucial? Environmental variables, stored in .env files, facilitate seamless integration of sensitive data throughout your application. The .env file provides a structured and secure way to manage environment variables, ensuring that sensitive data is not hard-coded into the source code. Discovering the Power of .env Files in Python: A Comprehensive Guide
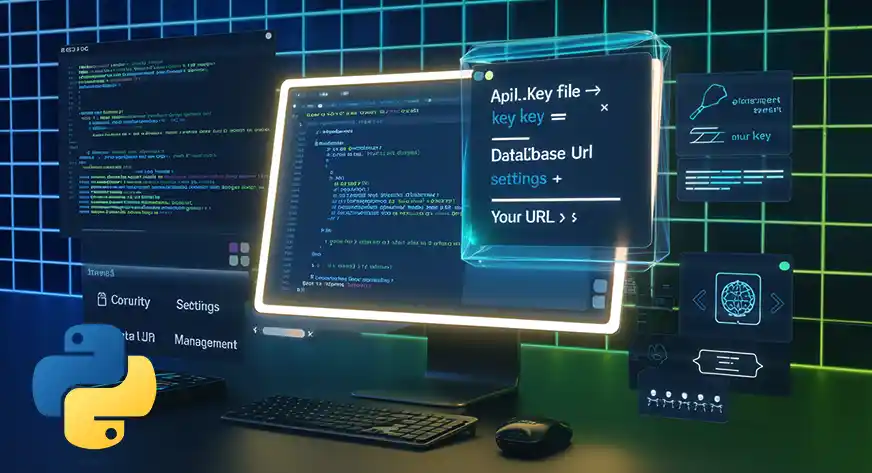
In Python, a `.env` file is used to store environment variables that are specific to a particular project. It allows you to separate your sensitive data from your code and keep it secure by not committing the configuration details into version control systems like GitHub. This approach helps maintain the integrity of your application and keeps its secrets confidential.
A `.env` file is a simple text-based configuration file that stores key-value pairs of environment variables. Records such as these are widely utilized for storing sensitive data like API keys, database credentials, and software settings. By leveraging .env files, developers can efficiently isolate sensitive information from their codebase, thereby streamlining management across diverse environments such as development, staging, and production.
What’s the purpose of employing dot env recordsdata in Python programming?
- Preserves sensitive data within your proprietary code repository.
- Facilitates seamless sharing of configurations across diverse platforms and mediums.
- Streamlines management of diverse environments without modifying code.
- Streamlines complex configuration settings into a logical and easily accessible framework.
What are .env files?
To get started with your Laravel application, you need to create a new file named `.env` in the root directory of your project. This file contains all environment-specific variables and settings that are used by Laravel during runtime.
In this step, you will add some basic configuration options and database credentials to your .env file.
Create an environment file named `.env` in the project’s root directory to define and store sensitive information such as API keys, database credentials, or other configuration settings for ease of use and security. Please append your customized settings to this configuration file.
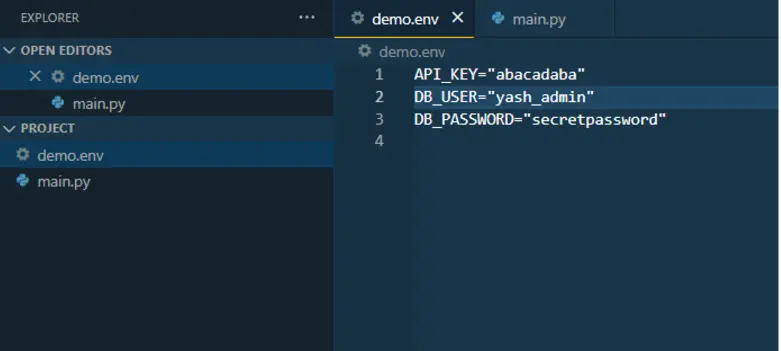
The python-dotenv library makes it simple to load environment variables from a .env file. To set this up, you can add the following lines of code to your Python script:
The Python-dotenv library is a popular alternative for loading .env files into Python. Set up it utilizing
pip set up python-dotenv
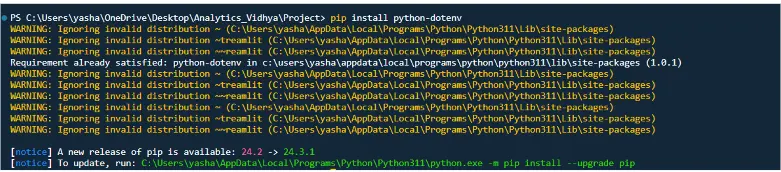
The application loads variables from a .env file. This process occurs in Step 3 of the deployment script. The .env file typically contains sensitive information such as database credentials and API keys, which are used to configure the environment. By loading these variables into the application, you can easily switch between different environments (like development, staging, or production) without having to update your code.
Loaded environment variables from .env file using python-dotenv.
The trail of the .env file using the load_dotenv() technique can be pinpointed through importing the necessary module from python-dotenv library and invoking it with the path to your .env file.
E.g. load_dotenv(:C/initiatives)
import os from dotenv import load_dotenv load_dotenv() api_key = os.environ.get('API_KEY', '') person = os.environ.get('DB_USER', '') password = os.environ.get('DB_PASSWORD', '') if api_key: print(f"Your API secret is: {api_key}") if person: print(f"Person is: {person}") if password: print(f"Password is: {password}")
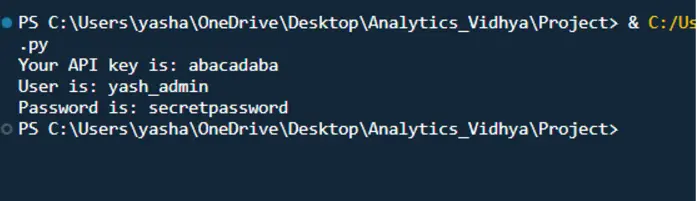
Utilize `.env` recordsdata securely by adhering to finest practices: Always load environment variables locally in your development surroundings and test their impact before deploying to production. Implement a secure strategy to store and handle sensitive data, like API keys and database credentials.
- Don’t commit sensitive data – add `.env` to your `.gitignore`!
- Ensure consistent naming conventions for variables to facilitate readability and maintainability of code?
- When relying on environment variables (.env files) instead of hardcoded fallback values, ensure the confidentiality of sensitive data is maintained effectively.
- Collaborate on design patterns without sensitive data exposure by sharing standardized files that outline expected structures and formats, ensuring transparency and consistency among team members.
Conclusion
Utilizing .env files in Python is a best practice for securely managing sensitive data, such as API keys, database credentials, and other configuration settings. Using the python-dotenv library, developers can seamlessly integrate sensitive data into their projects, ensuring a robust distinction between confidential information and their codebase.
This methodology optimizes safety, simplifies portability, and enables effortless configuration across diverse settings, including development, staging, and production environments. Bestowing adherence to established guidelines, such as omitting sensitive `.env` files from version control, utilizing informative variable labels, and providing a `.env.example` template, can notably simplify collaboration and minimize the likelihood of compromising confidential data.
Regardless of whether you’re building a small project or a large-scale software, seamlessly integrating .env files into your workflow guarantees a structured and secure approach for managing project configurations.
Are you searching for a comprehensive online Python course?
Regularly Requested Questions
Ans. A .env file serves as a secure repository for storing environment variables like API keys, database credentials, and other sensitive data. Keeping data isolated from production code improves security and collaboration.
Ans. ENV files commonly store sensitive information such as confidential passwords or API credentials. Together with them, exposing sensitive data to unauthorised customers could potentially compromise our model management’s integrity and confidentiality? The .gitignore file should contain the following line:
*.env
Ans. The python-dotenv library simplifies the process of loading environment variables from a .env file into your Python application. It streamlines management of atmospheric variables, minimizing the risk of inadvertently embedding sensitive information through hardcoded values.