For each Python programmer, whether or not within the area of information science and machine studying or software program growth, Python slicing operations are one of the vital environment friendly, versatile, and highly effective operations. Python slicing syntax permits the extraction and modification of information buildings like Lists, Strings, Tuples, Arrays, Pandas DataFrames, and even byte sequences. Whether or not we’ve to extract a piece of listing slicing in Python, manipulate characters utilizing string slicing, or simply wish to streamline the workflow, slicing presents a concise method to work with knowledge with out the usage of complicated loops or handbook indexing. On this Python slicing tutorial, we’ll dive deeper into how the Python slicing operations work and discover ways to use them successfully in packages and workflows.
What Are Slicing Operations in Python?
Slicing means reducing. Equally, in Python, it means accessing or extracting a sub-sequence (portion) of a sequence (like strings, lists, tuples, or arrays) by specifying a spread of indices. Slicing operations in Python contain utilizing colon operators [:] throughout the sq. brackets. The essential syntax entails:
[START: END: STEP]
START: Begin is the index from the place the slicing begins.
END: Finish is the index level as much as which the operation shall be carried out, i.e., it’s not included within the
operation.
STEP: Step is the increment index. Its default worth is 1, which suggests the entire sequence would be the output. If step=2, then each alternate worth shall be printed.
Why Use Slicing?
Slicing is a vital methodology because it permits us to entry and manipulate the information concisely, making the code extra readable and versatile throughout knowledge buildings. For instance:
Iterating with out Slicing
lst = [1, 2, 3, 4, 5] sublist = [] for i in vary(1, 4): sublist.append(lst[i]) print(sublist)
Iterating with Slicing
lst = [1, 2, 3, 4, 5] sublist = lst[1:4] print(sublist)
Output: [2, 3, 4]
Slicing Strategies in Python
Mainly, Python presents 2 alternative ways of slicing. One is [start: end: step] and the opposite is the .slice(begin, cease, step) operate. On this part, first we’ll undergo the syntax of those slicing operations, after this we’ll discover the key forms of slicing that we are able to carry out in Python.
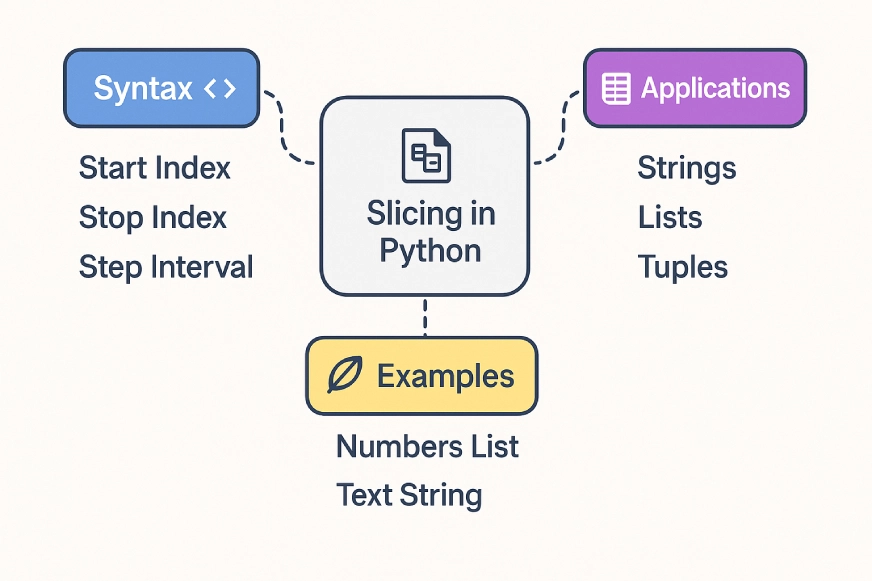
- Utilizing [start: end: step]
That is the most typical method to carry out the slicing operation on totally different components of the enter sequence.
Slicing with index [:] Examples
mixed_data = [10, "apple", 3.14, "banana", 42, "cherry"] # Slice from index 1 to 4 print(mixed_data[1:4]) print(20*"--") # Slice the listing from the begin to index 3 print(mixed_data[:3]) print(20*"--") # Slice each 2nd factor print(mixed_data[::2])
![Slicing with index [:] Examples](https://cdn.analyticsvidhya.com/wp-content/uploads/2025/05/img_2.webp)
- Utilizing Python slice() operate
The slice operate permits you to create a slice object, which shall be utilized to the sequence. This helps whenever you wish to retailer the slice specs and apply them to a number of sequences.
Syntax of .slice()
slice(begin, cease, step)
begin: The beginning index of the slice (inclusive).
cease: The stopping index of the slice (unique).
step: The step or stride (how a lot to increment the index after every step, non-obligatory).
Slicing with slice(). Examples
textual content = "Hey, world!" # Create a slice object to get the primary 5 characters s = slice(0, 5) # Apply the slice object to the string print(textual content[s]) # Output: "Hey"
Slicing each third factor
mixed_data = [10, "apple", 3.14, "banana", 42, "cherry"] s = slice(None, None, 3) # Apply the slice object to the listing print(mixed_data[s]) # Output: [10, 'banana']
Slice from index 2 to the top
s = slice(2, None) # Apply the slice object to the listing print(mixed_data[s]) # Output: [3.14, 'banana', 42, 'cherry']
Slice in reverse order
s = slice(None, None, -1) # Apply the slice object to the listing print(mixed_data[s]) # Output: ['cherry', 42, 'banana', 3.14, 'apple', 10]
Now we’ll look into the key forms of slicing operations in Python
1. Fundamental Slicing
Fundamental slicing refers to extracting a subsequence for knowledge sorts like string, listing, or tuple utilizing syntax [start: end: step]. It’s a basic instrument in Python that enables us to retrieve the subsequences simply. It additionally works with quite a lot of knowledge sorts, making it a flexible approach.
numbers = [10, 20, 30, 40, 50, 60] # Slice from index 1 to index 4 (unique) print(numbers[1:4]) # Output: [20, 30, 40]
textual content = "Hey, world!" # Slice from index 7 to index 12 (unique) print(textual content[7:12]) # Output: "world"
numbers_tuple = (1, 2, 3, 4, 5, 6) # Slice from index 2 to index 5 (unique) print(numbers_tuple[2:5]) # Output: (3, 4, 5)
2. Omitting ‘begin’, ‘cease’, or ‘step’
Omitting begin, cease, and step in slicing permits customers to make use of default values.
- Omitting begin defaults to the start of the sequence.
- Omitting cease means the slice goes till the top.
- Omitting step defaults to a step of 1.
Eradicating these components makes the code extra concise and versatile. It lets you create dynamic and generalized slicing with out explicitly defining the entire parameters.
Modifying Lists with Slicing
numbers = [10, 20, 30, 40, 50, 60] # Omitting begin, slice from the start to index 4 (unique) print(numbers[:4]) # Output: [10, 20, 30, 40] # Omitting cease, slice from index 2 to the top print(numbers[2:]) # Output: [30, 40, 50, 60]
Empty Slicing
numbers_tuple = (1, 2, 3, 4, 5, 6) # Omitting begin and step, slice the entire tuple print(numbers_tuple[:]) # Output: (1, 2, 3, 4, 5, 6)
Delete Parts
numbers = [2,4,5,12,64,45] numbers[1:4] = [] print(numbers) # Output: [2,64,45]
3. Damaging Slicing
Damaging indexing permits counting from the top of a sequence. In detrimental indexing, -1 refers back to the final factor, and -2 refers back to the second final. It helps when you have to entry components from the top of a sequence.
Accessing the Final Parts
numbers = [10, 20, 30, 40, 50, 60] # Slice on the final index print(numbers[-1]) # Output: [60]
Reversing a String
original_string = "howdy" reversed_string = original_string[::-1] print(reversed_string) # Output: "olleh"
4. Slicing Utilizing Step
The step parameter permits for specifying the interval between the weather, making it helpful when processing or sampling knowledge. A detrimental step can reverse the sequence simply, as seen above, making it very simple and handy to reverse the entire knowledge.
Slicing Each 2nd Component
numbers = [10, 20, 30, 40, 50, 60] # Slice with step 2, selecting each second factor print(numbers[::2]) # Output: [10, 30, 50]
Complicated Step Habits
numbers = [10, 20, 30, 40, 50, 60] # Slice finally index print(numbers[::-3]) # Output: [60,30]
5. Slicing with None
In Slicing, None can be utilized to signify the default worth for begin, cease, and finish. Utilizing None permits extra flexibility and readability within the programming. It’s a method to apply default slicing behaviour with out defining them manually.
Omitting utilizing None
numbers = [10, 20, 30, 40, 50, 60] # Slice each 2nd factor utilizing slice(None, None, 2) s = slice(None, None, 2) print(numbers[s]) # Output: [10, 30, 50]
6. Out-Of-Certain Slicing
Whenever you attempt to slice a sequence past its bounds (both with massive indices or with -ve indices out of vary), it gained’t elevate any error in Python and easily return the biggest legitimate slice with out worrying in regards to the exceptions.
numbers = [10, 20, 30, 40, 50, 60] # Slice past the size of the listing print(numbers[4:15]) # Output: [50, 50]
Slicing Past Size
textual content = "Hey, world!" # Slice past the size print(textual content[15:55]) # Output: no output
7. NumPy Array Slicing
In NumPy, slicing additionally works equally to the Python fundamental slicing. Additionally, NumPy is particularly designed for scientific computing and in addition permits sooner knowledge manipulation. This aids in additional supporting extra superior and environment friendly operations for giant datasets. Slicing permits NumPy to entry sub-arrays and in addition modify them effectively (i.e., permitting us to change the subarrays).
Slicing via 1-D Arrays
import numpy as np # Create a 1-D NumPy array arr = np.array([10, 20, 30, 40, 50, 60]) # Slice from index 1 to index 4 (unique) print(arr[1:4]) # Output: [20 30 40]
Like the essential slicing, it permits us to slice via the array from index 1 to 4 (unique), identical to the common Python Slicing. It additionally permits to carry out all the opposite operations mentioned above, in arrays as nicely.
# Slice each second factor from the array print(arr[::2]) # Output: [10 30 50]
Slicing via Multi-dimensional Arrays
# Create a 2-D NumPy array (matrix) arr_2d = np.array([[10, 20, 30], [40, 50, 60], [70, 80, 90]]) # Slice from row 1 to row 2 (unique), and columns 1 to 2 (unique) print(arr_2d[1:2, 1:3]) # Output: [[50 60]]
8. Pandas DataFrame Slicing
Pandas DataFrames are 2-dimensional labelled knowledge buildings that additionally help slicing operations. It permits slicing via the information factors via .loc() and .iloc(). Together with this, Pandas additionally helps boolean indexing.
Slicing the dataframe itself permits for filtering and manipulating massive datasets effectively. It permits to pick out subsets of information utilizing situations, making it a beneficial instrument for knowledge evaluation and machine studying.
Slicing utilizing Row Index (.iloc)
import pandas as pd # Create a DataFrame df = pd.DataFrame({ 'A': [1, 2, 3, 4, 5], 'B': [10, 20, 30, 40, 50] }) print(df) # Output # A B # 0 1 10 # 1 2 20 # 2 3 30 # 3 4 40 # 4 5 50 # Slice the primary three rows (unique of the fourth row) print(df.iloc[:3]) # A B # 0 1 10 # 1 2 20 # 2 3 30
Right here, the .iloc(3) slices the primary 3 rows(index 0 to 2) of the DataFrame.
Slicing utilizing Column Title (.loc)
# Create a DataFrame df = pd.DataFrame({ 'A': [1, 2, 3, 4, 5], 'B': [10, 20, 30, 40, 50] }) print(df) # Output # A B # 0 1 10 # 1 2 20 # 2 3 30 # 3 4 40 # 4 5 50 print(df.loc[df['A'] > 2]) # Output: # A B # 2 3 30 # 3 4 40 # 4 5 50
The .loc permits slicing the labels with column names or as an index as False. Right here, we’re slicing primarily based on the situation that the column ”A” worth should be higher than 2.
9. Byte Sequence Slicing
Python presents byte sequences akin to bytes and bytearray, which help slicing identical to lists, strings, or arrays. Byte sequence comes into the image after we are utilizing the binary knowledge sorts, and slicing permits you to extract related components of binary knowledge with ease and effectivity.
Slicing a byte Object
byte_seq = b'Hey, world!' # Slice from index 0 to index 5 (unique) print(sort(byte_seq)) print(byte_seq[:5]) # Output: , b'Hey'
Slicing a bytearray(Mutable bytes)
byte_arr = bytearray([10, 20, 30, 40, 50, 60]) # Slice from index 2 to index 5 (unique) print(byte_arr[2:5]) # Output: bytearray(b'2x1e
Right here, the output is in values equivalent to ASCII characters. This occurs when the output shouldn’t be within the printable vary. So, bytearray(b’2x1e
print(listing(byte_arr[2:5])) # Output: [30, 40, 50] # Ouput: [30,40,50]
Advantages of Utilizing Slicing in Python
There are numerous advantages of utilizing slicing operations in Python, together with:
- Effectivity: Slicing permits quick entry of the specified sub-sequence out of bigger datasets without having to loop.
- Conciseness: It permits extra concise and readable code for knowledge manipulation.
- Flexibility: With the assistance of libraries like NumPy and Pandas, slicing via multidimensional knowledge provides an environment friendly means for knowledge preprocessing.
- Reminiscence Environment friendly: Slicing is optimized for efficiency. Additionally, Python’s inner mechanism ensures that slicing operations are quick and reminiscence environment friendly, in contrast to handbook indexing, which takes plenty of handbook coding and will increase reminiscence utilization.
Issues to Keep away from Whereas Utilizing Slicing Operations
Right here are some things to keep away from whereas utilizing slicing operations in Python.
- Exceeding Index Boundaries: Slicing past the sequence size won’t trigger any error in Python. Nonetheless, this can lead to undesirable outcomes, particularly when working with bigger datasets.
- Complicated Indexing and Slicing Syntax: The slicing syntax entails sequence[start : stop: end], however choosing the index/place of the factor we would like will even give the specified factor.
- Slicing With out Contemplating Mutability: Slicing can be utilized to change the sequence, however to make use of this, one should contemplate whether or not the information sort they’re coping with helps mutability or not. For instance, lists and byte arrays are mutable; then again, strings, tuples, and bytes are immutable. To allow them to’t be modified straight via slicing.
- Slicing with Invalid Step Values: Whereas utilizing slicing, one should additionally contemplate the step as it’s going to determine what number of factors shall be skipped in between. However the usage of an invalid worth can result in surprising outcomes, inflicting inefficient operations.
Conclusion
Slicing in Python is an environment friendly and highly effective means that permits you to effectively entry and manipulate the Python knowledge sorts like Lists, strings, tuples, NumPy arrays, and Pandas DataFrames. So, whether or not you’re slicing a listing, working with multi-dimensional arrays in NumPy, or coping with massive datasets utilizing Pandas, slicing all the time gives a transparent and concise method to work with sequences. By mastering slicing, one can write cleaner and extra environment friendly code, which is crucial for each Python programmer.
Login to proceed studying and revel in expert-curated content material.